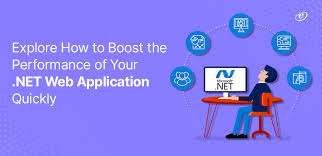
In todayโs fast-paced digital landscape, the performance of web applications plays a critical role in user satisfaction and business success. ASP.NET Development has long been a preferred choice for building robust, scalable web applications, but ensuring optimal performance requires a strategic approach.
This guide delves into practical techniques to optimize ASP.NET applications, helping you deliver faster, more efficient solutions that meet modern user expectations.
Why Performance Optimization Matters
The performance of your ASP.NET application directly impacts user experience, search engine rankings, and operational costs. Slow-loading applications can lead to higher bounce rates and lost revenue. By optimizing your application, you can:
- Enhance User Satisfaction: Faster applications improve engagement and retention.
- Boost Scalability: Handle higher traffic loads efficiently.
- Reduce Costs: Minimize server resource usage and operational expenses.
1. Use Asynchronous Programming
Asynchronous programming allows your application to handle multiple tasks concurrently, improving responsiveness and resource utilization.
How to Implement Asynchronous Programming in ASP.NET
- Use async and await keywords for non-blocking operations.
- Replace synchronous calls with asynchronous equivalents, such as HttpClient.GetAsync instead of WebClient.DownloadString.
This approach is particularly effective for I/O-bound tasks, such as database queries and API calls.
2. Optimize Database Queries
Inefficient database interactions are a common bottleneck in web applications. To improve performance:
- Use Stored Procedures: Precompiled queries reduce execution time.
- Optimize Indexing: Ensure your database tables have appropriate indexes.
- Minimize Data Retrieval: Fetch only the necessary data using SELECT statements with specific columns.
- Implement Caching: Store frequently accessed data in memory to reduce database load.
3. Leverage Caching Techniques
Caching is a powerful way to enhance application performance by reducing redundant processing. ASP.NET provides several caching options:
- Output Caching: Store the output of pages or controls to serve subsequent requests faster.
- Data Caching: Use in-memory storage for frequently used data.
- Distributed Caching: For large-scale applications, use caching solutions like Redis or Memcached.
Example of Output Caching
csharp
Copy code
[OutputCache(Duration = 60, VaryByParam = “none”)]ย ย
public ActionResult Index()ย ย
{ย ย
ย ย ย ย return View();ย ย
}ย ย
ย
4. Minimize HTTP Requests
Reducing the number of HTTP requests can significantly improve load times. Hereโs how:
- Combine Files: Merge CSS and JavaScript files.
- Use Bundling and Minification: ASP.NET provides tools to bundle and minify resources, reducing their size and the number of requests.
- Enable Compression: Use Gzip or Brotli to compress resources before sending them to the client.
5. Optimize Application Configuration
Your applicationโs configuration settings can affect its performance. Consider these best practices:
- Enable Release Mode: Ensure your application is compiled in release mode for production.
- Disable Debugging: Turn off debugging features to reduce overhead.
- Use Custom Error Pages: Avoid exposing sensitive information with detailed error messages.
Example: Configure Release Mode in Web.config
xml
Copy code
<system.web>ย ย
ย ย ย ย <compilation debug=”false” targetFramework=”4.7.2″ />ย ย
</system.web>ย ย
ย
6. Implement Load Balancing
For high-traffic applications, load balancing distributes incoming requests across multiple servers, ensuring consistent performance.
Benefits of Load Balancing
- Prevents server overload.
- Improves application availability and reliability.
- Enhances scalability for growing traffic demands.
7. Monitor and Profile Your Application
Regularly monitoring your application helps identify and address performance issues proactively.
Tools for Monitoring ASP.NET Applications
- Application Insights: Tracks performance metrics and diagnostics.
- dotTrace: Profiles your application to identify slow code paths.
- ELMAH: Logs errors and exceptions for analysis.
8. Use Content Delivery Networks (CDNs)
CDNs distribute static resources like images, CSS, and JavaScript across multiple servers worldwide. This reduces load times by serving content from servers closest to the user.
Benefits of CDNs
- Faster content delivery.
- Reduced server load.
- Improved user experience for global audiences.
9. Optimize Session Management
Session management can impact performance, especially for large-scale applications. To optimize sessions:
- Use Session State Wisely: Store minimal data in session variables.
- Enable Out-of-Process Storage: Use distributed session state providers like SQL Server or Redis.
10. Upgrade to the Latest ASP.NET Version
Microsoft continuously improves ASP.NET with performance enhancements and new features. Ensure your application is running on the latest version to take advantage of these improvements.
Conclusion: Unlock the Full Potential of ASP.NET Development
Optimizing your ASP.NET application for performance is a continuous process that requires attention to detail and strategic planning. By implementing these techniques, you can deliver faster, more reliable applications that meet user expectations and business goals.
Looking for expert assistance in ASP.NET development? Partner with Adequate Infosoft to unlock the full potential of your web applications. Contact us at +91-120-4198878 to discuss your project today!ย
Leave a Reply